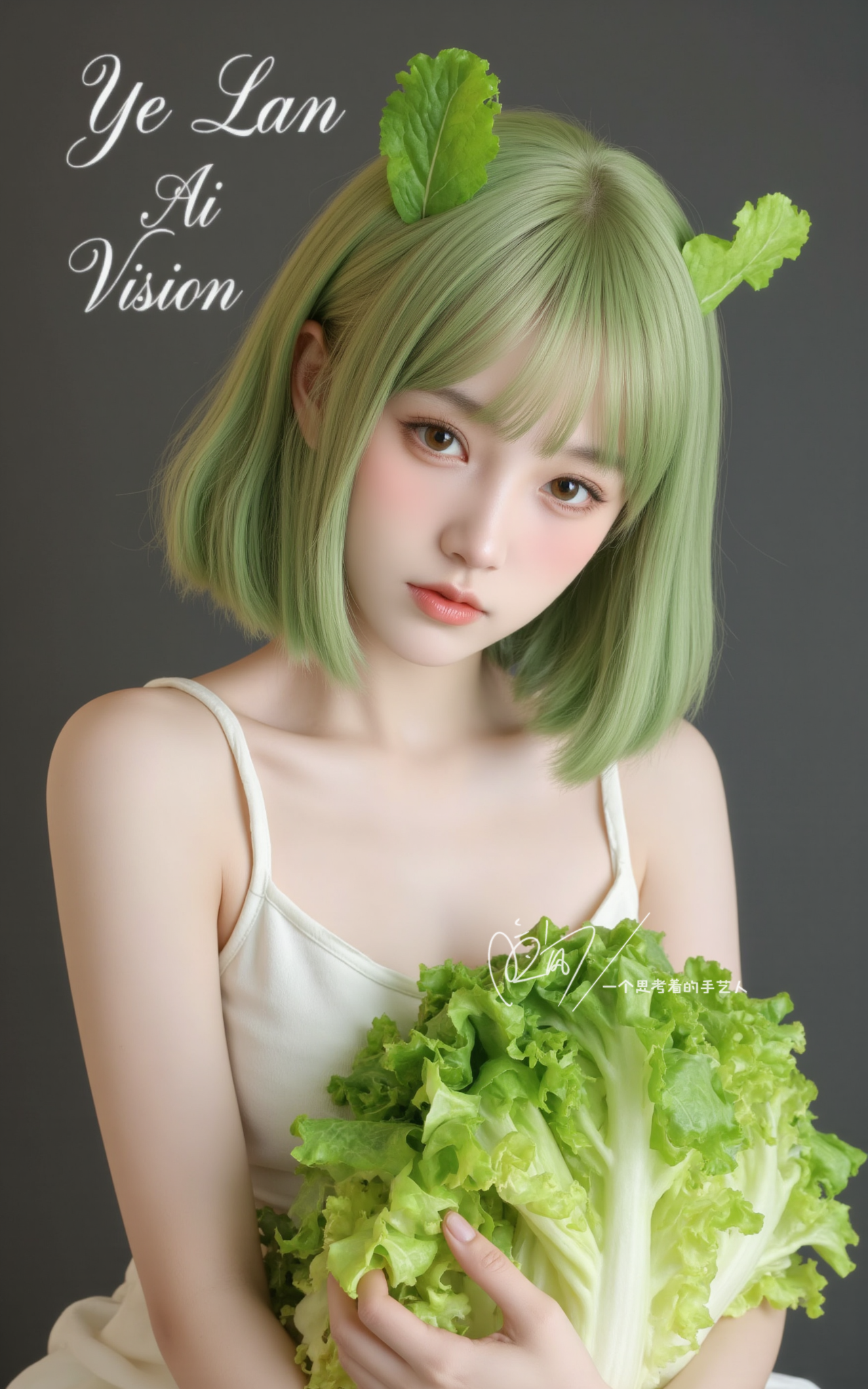
点餐系统(比喻)
好的,我来用生动的比喻重新注释这些代码。想象这是一个餐厅点餐系统:
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>郭大爷ComfyUI图像生成器</title>
<script>
// 点餐函数:就像顾客告诉服务员要点什么菜
async function generateImage() {
// 获取顾客的点单内容(提示词)
const promptText = document.getElementById("prompt-text").value;
try {
// 服务员把订单送到厨房(发送API请求)
const response = await fetch('/generate_image', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ prompt_text: promptText })
});
// 检查厨房接单状态
const result = await response.json();
if (result.status === 'success') {
alert("厨师已经开始准备了,请稍等..."); // 告诉顾客等待
setTimeout(fetchLatestImage, 5000); // 5秒后去看菜好了没
} else {
alert("点单失败:" + result.message);
}
} catch (error) {
alert("服务员跑单了:" + error.message);
}
}
// 查看菜品是否准备好:就像服务员去厨房看菜好了没
async function fetchLatestImage() {
try {
const response = await fetch('/get_latest_image');
if (response.ok) {
// 菜品准备好了,端给顾客看
const imageBlob = await response.blob();
const imageUrl = URL.createObjectURL(imageBlob);
document.getElementById('generated-image').src = imageUrl;
} else {
const result = await response.json();
alert("菜还没好:" + result.message);
}
} catch (error) {
alert("服务员摔倒了:" + error.message);
}
}
</script>
</head>
<body>
<!-- 餐厅的点餐界面 -->
<h1>ComfyUI 图像生成器</h1>
<div>
<!-- 点餐单 -->
<label for="prompt-text">输入提示词:</label>
<input type="text" id="prompt-text" placeholder="请输入提示词">
</div>
<!-- 下单按钮 -->
<button onclick="generateImage()">开始生成图像</button>
<div>
<!-- 上菜的托盘区域 -->
<h2>生成的图像:</h2>
<img id="generated-image" src="" alt="生成的图像将在此显示" style="max-width: 100%; height: auto;">
</div>
</body>
</html>
from flask import Flask, render_template, request, jsonify, send_file
import subprocess
import os
# 创建Flask应用实例 - 相当于开设一家餐厅
app = Flask(__name__)
# 处理点餐请求的API - 相当于服务员接单
@app.route('/generate_image', methods=['POST'])
def generate_image():
try:
# 获取顾客点的菜(提示词)
prompt_text = request.json.get("prompt_text", "default")
# 服务员把订单交给厨房(调用生成脚本)
# 就像把点菜单送到后厨
result = subprocess.run(
['python', 'C:/1/V2/websockets_api_example.py', prompt_text],
check=True,
capture_output=True,
text=True
)
# 告诉顾客已经开始准备了
return jsonify({"status": "success", "message": "Image generation started", "output": result.stdout})
except subprocess.CalledProcessError as e:
# 厨房出问题了(执行脚本出错)
error_message = e.stderr or str(e)
print("厨房着火了:", error_message)
return jsonify({"status": "error", "message": "Failed to start image generation", "error": error_message}), 500
except Exception as e:
# 其他意外情况
print("服务员摔倒了:", str(e))
return jsonify({"status": "error", "message": str(e)}), 500
# 查看菜品是否准备好 - 相当于服务员去厨房看菜
@app.route('/get_latest_image', methods=['GET'])
def get_latest_image():
try:
# 检查厨房的出菜单(图片路径记录)
with open("latest_image.txt", "r") as f:
image_path = f.read().strip()
# 如果菜做好了,就端给顾客
if os.path.exists(image_path):
return send_file(image_path, mimetype='image/png')
else:
return jsonify({"status": "error", "message": "Image not found"}), 404
except FileNotFoundError:
# 还没开始做菜
return jsonify({"status": "error", "message": "No image generated yet"}), 404
# 餐厅门面 - 顾客进来看到的页面
@app.route('/')
def index():
return render_template('index.html')
# 开门营业
if __name__ == "__main__":
app.run(port=5000)
# 这个文件就像餐厅的后厨,负责实际的"烹饪"过程
import websocket #websocket-client库,就像厨房的基础设施
import uuid #生成订单号的工具
import json #菜品配方的格式化工具
import urllib.request #与主厨(ComfyUI)通信的工具
import urllib.parse
import os
import glob
import sys
# 设置厨房地址和订单编号
server_address = "127.0.0.1:8188" # 主厨的位置
client_id = str(uuid.uuid4()) # 生成唯一的订单号
def queue_prompt(prompt):
# 向主厨递交订单
# 就像把顾客的要求转化为厨师能理解的专业术语
request_data = {"prompt": prompt, "client_id": client_id}
data = json.dumps(request_data).encode('utf-8')
print("向主厨传达顾客要求:", request_data)
headers = {'Content-Type': 'application/json'}
req = urllib.request.Request(f"http://{server_address}/prompt", data=data, headers=headers)
try:
response = urllib.request.urlopen(req)
return json.loads(response.read())
except urllib.error.HTTPError as e:
print(f"主厨没听清楚: {e.code} - {e.reason}")
print(e.read().decode())
raise
def get_images(ws, prompt):
# 等待主厨完成菜品
# 就像在厨房门口等着看菜品进度
prompt_id = queue_prompt(prompt)['prompt_id']
output_images = {}
while True:
out = ws.recv()
if isinstance(out, str):
message = json.loads(out)
if message['type'] == 'executing':
data = message['data']
if data['node'] is None and data['prompt_id'] == prompt_id:
break #菜品完成了
else:
continue #还在准备中
return output_images
def generate_image_with_comfyui(prompt_text):
# 主要的烹饪流程
# 就像按照食谱准备一道菜
prompt = {
# ... (配方细节)
}
# 通知主厨开始制作
ws = websocket.WebSocket()
ws.connect(f"ws://{server_address}/ws?clientId={client_id}")
images = get_images(ws, prompt)
ws.close()
# 找到刚做好的菜品(图片文件)
output_directory = r"C:\AI\ComfyUI-mini\output"
list_of_files = glob.glob(os.path.join(output_directory, "GUO*.PNG"))
if not list_of_files:
print("菜品还没准备好")
return
# 记录最新完成的菜品位置
latest_file = max(list_of_files, key=os.path.getctime)
with open("latest_image.txt", "w") as f:
f.write(latest_file)
print(f"菜品已经准备完毕,放在: {latest_file}")
# 程序入口 - 厨房开工
if __name__ == "__main__":
if len(sys.argv) > 1:
prompt_text = sys.argv[1] # 获取顾客的具体要求
generate_image_with_comfyui(prompt_text)
else:
print("顾客还没点菜呢")
这样的比喻是不是更容易理解了?整个系统就像一个餐厅:
- 前端(index.html)就是餐厅的点餐界面
- web_app.py就是服务员,负责接待顾客、传递订单
- websockets_api_example.py就是后厨,负责实际的"烹饪"(图片生成)过程
- ComfyUI就是主厨,实际执行图片生成的核心
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 兰雀AI