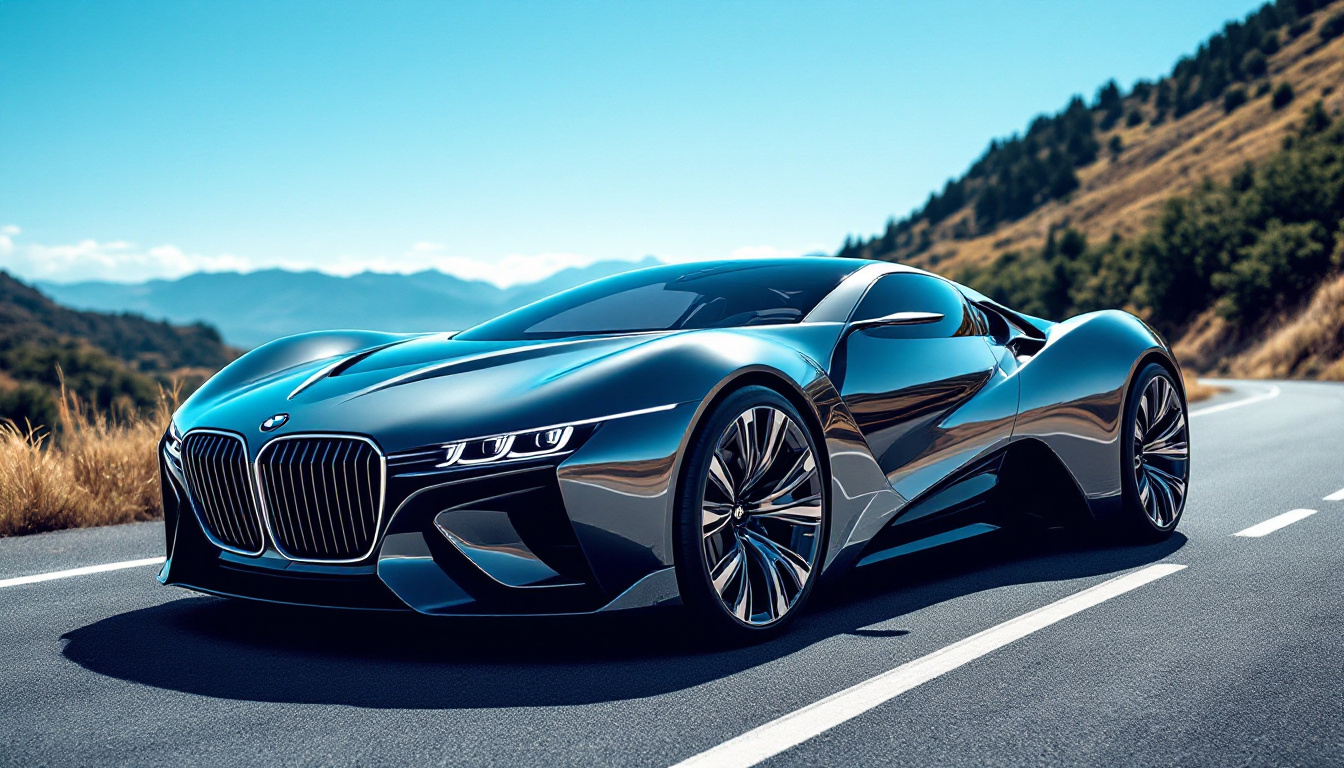
Python程序打包为EXE
Python程序打包为EXE的开发文档
1. 项目准备
1.1 环境准备
首先确保安装了所需的包:
# 安装pip包管理器的国内镜像源
pip config set global.index-url https://pypi.tuna.tsinghua.edu.cn/simple
# 安装必要的包
pip install pyinstaller
pip install requests
pip install flask
pip install flask-cors
1.2 项目结构
V5/
├── test_port.py # 主程序
├── build_exe.py # 打包脚本
├── web_app.py # Web应用(如果有)
└── mock_comfyui.py # 模拟服务(如果有)
2. 开发流程
2.1 编写主程序(test_port.py)
import socket
import requests
import time
def test_port(host, port, description):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.settimeout(3) # 设置超时时间
try:
result = sock.connect_ex((host, port))
if result == 0:
print(f"✓ {description}已开放")
else:
print(f"✗ {description}未开放")
except socket.error as e:
print(f"✗ {description}测试失败: {e}")
finally:
sock.close()
def test_flask_service(port=80):
try:
response = requests.get(f'http://localhost:{port}', timeout=3)
if response.status_code == 200:
print(f"✓ 端口{port}的Flask服务运行正常")
else:
print(f"✗ 端口{port}的Flask服务返回状态码: {response.status_code}")
except Exception as e:
print(f"✗ 端口{port}的Flask服务测试失败: {str(e)}")
if __name__ == "__main__":
print("=== 端口测试工具 ===")
print("正在进行端口测试...\n")
test_port('127.0.0.1', 80, "本地80端口")
test_port('127.0.0.1', 8188, "本地8188端口(ComfyUI)")
test_port('150.138.73.158', 80, "远程80端口")
test_port('150.138.73.158', 8188, "远程8188端口(ComfyUI)")
print("\n正在测试服务状态...")
test_flask_service(80)
test_flask_service(8188)
print("\n测试完成!")
print("按Enter键退出...")
input() # 等待用户按键后退出
2.2 编写打包脚本(build_exe.py)
import PyInstaller.__main__
PyInstaller.__main__.run([
'test_port.py',
'--onefile',
'--name=端口测试工具',
'--icon=test.ico' # 如果有图标文件的话
])
3. 打包步骤
3.1 测试主程序
# 进入项目目录
cd V5
# 测试主程序是否正常运行
python test_port.py
3.2 执行打包
# 方法1:使用打包脚本
python build_exe.py
# 方法2:直接使用pyinstaller命令
pyinstaller --onefile --name "端口测试工具" test_port.py
3.3 查找生成的exe
打包完成后,在 dist
文件夹中找到生成的exe文件:
V5/dist/端口测试工具.exe
4. 常用PyInstaller参数说明
--onefile
: 打包成单个exe文件--name
: 指定生成的exe文件名--noconsole
: 不显示控制台窗口(GUI程序用)--icon
: 指定程序图标--add-data
: 添加额外数据文件--hidden-import
: 添加隐式导入的模块
5. 常见问题解决
5.1 找不到模块
确保所有需要的包都已安装:
pip install -r requirements.txt # 如果有requirements.txt
5.2 运行时缺少DLL
可能需要添加额外的依赖:
pip install pywin32
5.3 打包后运行报错
尝试在打包命令中添加 --debug all
参数查看详细错误信息:
pyinstaller --onefile --debug all test_port.py
6. 下次开发流程
- 准备Python源代码
- 安装必要的包
- 编写build_exe.py
- 测试源代码
- 执行打包
- 测试生成的exe
这样,下次开发其他软件时,只需要:
- 发送这个文档给我
- 说明新软件的需求
- 我会帮你完成相应的开发和打包过程
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 兰雀AI