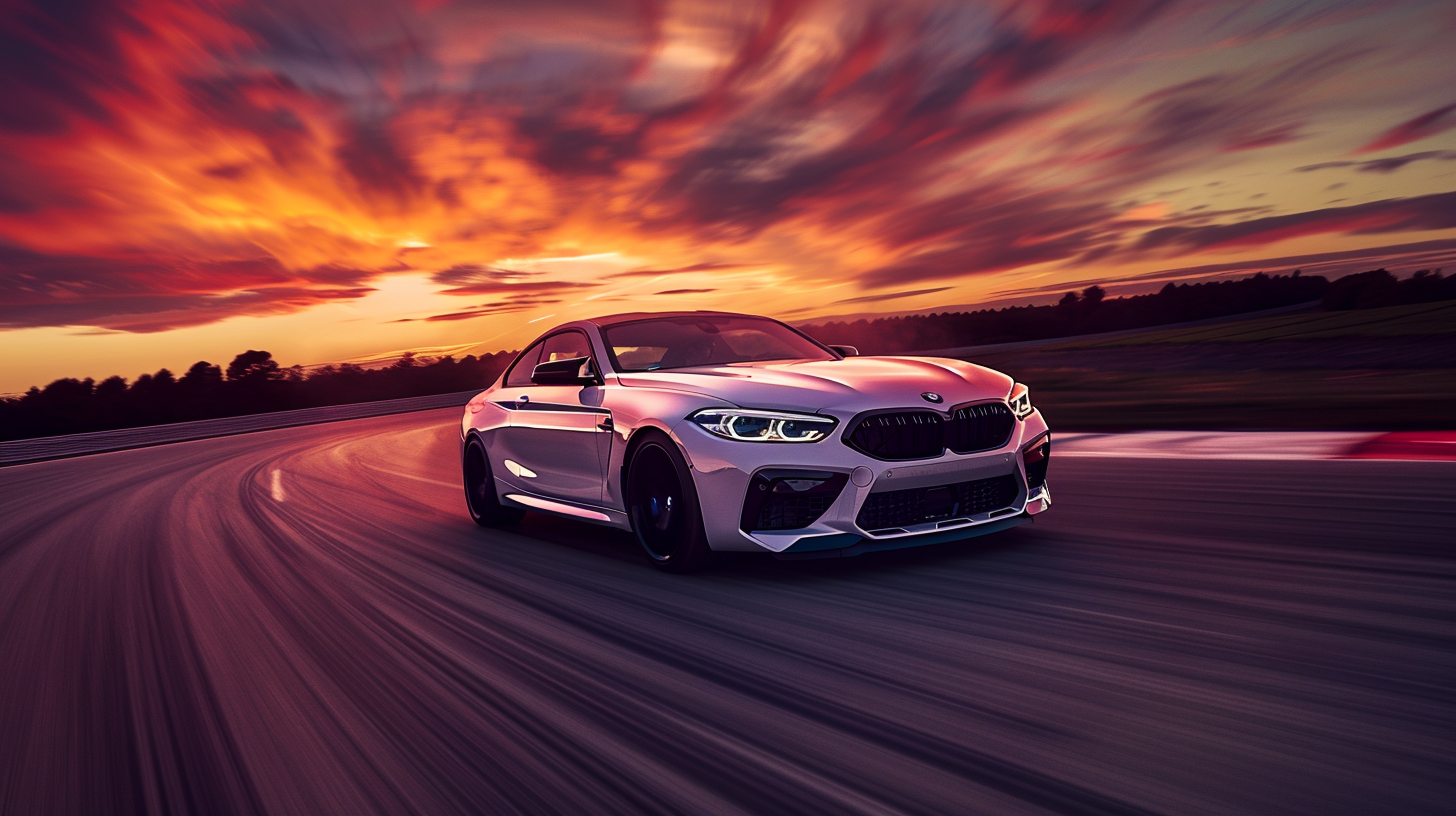
文生图API
好的,以下是修改后的完整的 index.php
和 api.php
文件内容。你可以直接用它们覆盖你现有的文件。
1. index.php
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>文生图 API 调用示例</title>
</head>
<body>
<h1>文生图生成</h1>
<label for="prompt">输入提示词:</label>
<input type="text" id="prompt" placeholder="例如:一位年轻女子,穿着绿色棒球帽">
<button id="generate">生成图像</button>
<div id="result"></div>
<script>
async function generateImage() {
const prompt = document.getElementById('prompt').value;
const response = await fetch('api.php', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ prompt: prompt })
});
const result = await response.json();
if (result.code === 0 && result.data.generateUuid) {
// 如果生成成功,开始查询结果
document.getElementById('result').innerText = '正在查询生成结果...';
await queryImage(result.data.generateUuid);
} else {
document.getElementById('result').innerText = JSON.stringify(result, null, 2);
}
}
// 查询生成结果的函数
async function queryImage(generateUuid) {
while (true) {
const response = await fetch('api.php', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ generateUuid: generateUuid })
});
const result = await response.json();
if (result.code === 0) {
const images = result.data.images || [];
if (images.length > 0) {
let imageUrls = images.map(image => `<img src="${image.imageUrl}" alt="生成的图像" style="max-width: 200px; margin: 5px;">`).join('');
document.getElementById('result').innerHTML = imageUrls;
break;
} else {
const generateStatus = result.data.generateStatus;
document.getElementById('result').innerText = `图像状态:${generateStatus},图像正在生成中...`;
}
} else {
document.getElementById('result').innerText = JSON.stringify(result, null, 2);
break;
}
// 等待一段时间再查询
await new Promise(resolve => setTimeout(resolve, 5000)); // 5 秒
}
}
// 绑定按钮点击事件
document.getElementById('generate').addEventListener('click', generateImage);
</script>
</body>
</html>
2. api.php
<?php
header('Content-Type: application/json');
// API 配置
$accessKey = '替换为你的 AccessKey'; // 你的 AccessKey
$secretKey = '替换为你的 SecretKey'; // 你的 SecretKey
// 获取请求方法
$requestMethod = $_SERVER['REQUEST_METHOD'];
if ($requestMethod === 'POST') {
// 获取请求体
$requestBody = json_decode(file_get_contents('php://input'), true);
if (isset($requestBody['prompt'])) {
$prompt = $requestBody['prompt'];
// 发起文生图请求
$timestamp = round(microtime(true) * 1000);
$nonce = bin2hex(random_bytes(5));
$uri = '/api/generate/webui/text2img';
$signature = generateSignature($uri, $timestamp, $nonce, $secretKey);
$requestData = [
'templateUuid' => '5d7e67009b344550bc1aa6ccbfa1d7f4',
'generateParams' => [
'prompt' => $prompt,
'imgCount' => 1,
]
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://openapi.liblibai.cloud/api/generate/webui/text2img?AccessKey=$accessKey&Signature=$signature&Timestamp=$timestamp&SignatureNonce=$nonce");
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($requestData));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response; // 返回文生图的响应
} elseif (isset($requestBody['generateUuid'])) {
// 查询结果
$generateUuid = $requestBody['generateUuid'];
// 发起查询请求
$timestamp = round(microtime(true) * 1000);
$nonce = bin2hex(random_bytes(5));
$uri = '/api/generate/webui/status';
$signature = generateSignature($uri, $timestamp, $nonce, $secretKey);
$queryData = [
'generateUuid' => $generateUuid,
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://openapi.liblibai.cloud/api/generate/webui/status?AccessKey=$accessKey&Signature=$signature&Timestamp=$timestamp&SignatureNonce=$nonce");
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($queryData));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response; // 返回查询结果
} else {
echo json_encode(['code' => 100051, 'msg' => '缺少必要参数']);
}
}
// 生成签名的函数
function generateSignature($uri, $timestamp, $nonce, $secretKey) {
$content = $uri . "&" . $timestamp . "&" . $nonce;
return rtrim(strtr(base64_encode(hash_hmac('sha1', $content, $secretKey, true)), '+/', '-_'), '=');
}
如何替换
- 将以上
index.php
和api.php
的内容分别复制到现有的文件中,覆盖原有内容。 - 在
api.php
中,将$accessKey
和$secretKey
替换为你自己的有效密钥。 - 保存修改并确保你的 Web 服务器正在运行。
- 通过访问
index.php
文件来测试新的功能。
这两个文件已经包含了生成图像和查询结果的完整逻辑,可以完成你要求的功能。如果你仍然有问题,欢迎随时询问!
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 兰雀AI